Ques 1: Consider the following C code:
GATE 2017 Q no 13
#include<stdio.h> int *assignval (int *x, int val) { *x = val; return x; } void main () { int *x = malloc(sizeof(int)); if (NULL == x) return; x = assignval (x,0); if (x) { x = (int *)malloc(sizeof(int)); if (NULL == x) return; x = assignval (x,10); } printf("%d\n", *x); free(x); }
The code suffers from which one of the following problems:
(A) compiler error as the return of malloc is not typecast appropriately.
(B) compiler error because the comparison should be made as x==NULL and not as shown.
(C) compiles successfully but execution may result in dangling pointer.
(D) compiles successfully but execution may result in memory leak.
Ans: (D) compiles successfully but execution may result in memory leak.
Solution: (A) In C++ we need to do typecasting. C does automatic implicit typecasting.
In C, int* ptr=malloc(sizeof(int)) is valid. C compiler happily converts void* to int*
(B) Null means address 0. if (a==0) OR if (0==a) There is no difference.
(C) Always x is pointing to a valid memory location. Dangling Pointer means if it points to a memory location which is deleted(freed). So no dangling pointer.
(D) x will loss the previous address it was pointing to. So it will result in memory leak.
Ques 2: Consider the following two functions.
GATE 2017 Q no 35
void fun1(int n) { if(n == 0) return; printf("%d", n); fun2(n - 2); printf("%d", n); } void fun2(int n) { if(n == 0) return; printf("%d", n); fun1(++n); printf("%d", n); }
The output printed when fun1(5) is called is
(A) 53423122233445
(B) 53423120112233
(C) 53423122132435
(D )53423120213243
Ans: (A) 53423122233445
Unroll recursion up to a point,where we can distinguish the given options
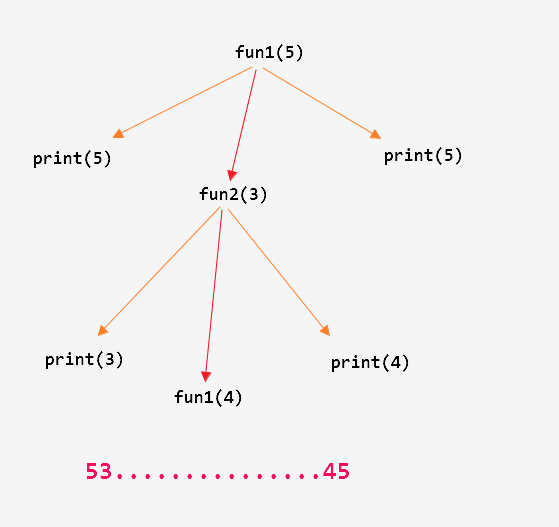
Ques 3: Consider the functions foo and bar given below.
GATE 2017 Q no 36
int foo(int val) { int x=0; while(val > 0) { x = x + foo(val--); } return val; }
int bar(int val) { int x = 0; while(val > 0) { x= x + bar(val-1); } return val; }
Invocations of foo(3) and bar(3) will result in:
(A) Return of 6 and 6 respectively.
(B) Infinite loop and abnormal termination respectively.
(C) Abnormal termination and infinite loop respectively.
(D) Both terminating abnormally.
Ans: (C) Abnormal termination and infinite loop respectively.
Solution: Consider foo. Initially val=3
foo(val−−) is equivalent to foo(val) and then val=val−1 i.e foo(3) and val=2
So,foo(3) calls foo(3) which in turn calls foo(3) this goes on
So, here we can see that foo(3) is called infinite number of times which causes memory overflow and abrupt termination
and one more thing to observe is infinite loop is not there since the val is decremented in the first iteration.
Consider bar. Here, we can see the val is not decrementing in the loop. So,
- bar(3) will call bar(2)
- bar(2) will call bar(1)
- bar(1) will call bar(0). Here, bar(0) return 0
- bar(1) will call bar(0)
- bar(1) will call bar(0)
This will go on so here there is a problem of infinite loop but not abrupt termination since it does not cause memory overflow.
Ques 4: Consider the following C Program
GATE 2017 Q no 53
#include<stdio.h> #include<string.h> void printlength(char *s, char *t) { unsigned int c=0; int len = ((strlen(s) - strlen(t)) > c) ? strlen(s) : strlen(t); printf("%d\n", len); } void main() { char *x = "abc"; char *y = "defgh"; printlength(x,y); }
Recall that strlen is defined in string.h as returning a value of type size_t, which is an unsigned int. The output of the program is _____ .
Ans: 3
Solution: (strlen(s)−strlen(t))=3−5=−2
But in C, when we do operation with two unsigned integers, result is also unsigned.
(strlen returns size_t which is unsigned in most systems).
So, this result “−2” is treated as unsigned and its value is INT_MAX−2
Now, the comparison is between this large number and another unsigned number c which is 0. So, the comparison return TRUE here.
Hence the conditional operator will return the true statement which is strlen(abc)=3
Ques 5: The output of executing the following C program is _______________ .
GATE 2017 Q no 55
#include<stdio.h> int total(int v) { static int count = 0; while(v) { count += v&1; v >>= 1; } return count; } void main() { static int x=0; int i=5; for(; i>0; i--) { x = x + total(i); } printf("%d\n", x); }
Ans: 23
Solution: // inside total()
while(v)
{
count += v&1; \\ check the lowest bit of v
v >>= 1; \\ or v = v >> 1 : right shift the bit pattern of v
}
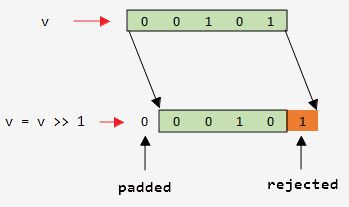
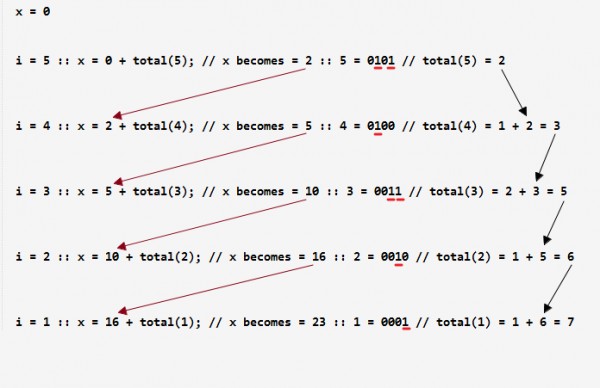
x= 2 + 3 + 5 + 6 + 7 = 23