Ques 1: What is printed by the following ANSI C program?
GATE 2022 Q no 21
#include<stdio.h> int main(int argc, char *argv[]) { int x = 1, z[2] = {10, 11}; int *p = NULL; p = &x; *p = 10; p = &z[1]; *(&z[0] + 1) += 3; printf("%d, %d, %d\n", x, z[0], z[1]); return 0; }
(A) 1 ,10 ,11
(B) 1 ,10 ,14
(C) 10 ,14 ,11
(D) 10 ,10 ,14
Ans: (D) 10 ,10 ,14
Solution: Initially p is holding the address of x
So, *p=10 will change the value of x to 10
After that p will hold the address of z[1]
*(&z[0] + 1) += 3 will change the contents of z[0] +1 i.e z[1] incremented by 3
But contents of z[0] remains unchanged
Finally, output will be 10, 10, 14
Ques 2: What is printed by the following ANSI C program?
GATE 2022 Q no 43
#include<stdio.h> int main(int argc, char *argv[]) { int a[3][3][3] = {{1, 2, 3, 4, 5, 6, 7, 8, 9}, {10, 11, 12, 13, 14, 15, 16, 17, 18}, {19, 20, 21, 22, 23, 24, 25, 26, 27}}; int i = 0, j = 0, k = 0; for( i = 0; i < 3; i++ ){ for(k = 0; k < 3; k++ ) printf("%d ", a[i][j][k]); printf("\n"); } return 0; }
(A) 1 2 3 10 11 12 19 20 21 | (B) 1 4 7 10 13 16 19 22 25 |
(C) 1 2 3 4 5 6 7 8 9 | (D) 1 2 3 13 14 15 25 26 27 |
Ans: (A)
1 2 3
10 11 12
19 20 21
Solution: a is 3D array with size [3][3][3]
Therefore each 2D array contains 9 elements, we have 3 such arrays
0th 2D array have {1,2,3,4,5,6,7,8,9}
1st 2D array have {10,11,12,13,14,15,16,17,18}
2nd 2D array have {19,20,21,22,23,24,25,26,27}
each 2D array is collection of 1D array.
We have 3 one dimensional arrays in one 2D
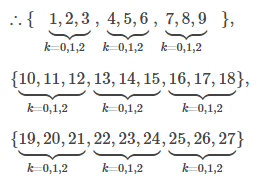
when i=0, j=0, output = {1,2,3}
when i=1, j=0, output = {10,11,12}
when i=2, j=0, output = {19,20,21}
Ques 3: What is printed by the following ANSI C program?
GATE 2022 Q no 44
#include<stdio.h> int main(int argc, char *argv[]){ char a = 'P'; char b = 'x'; char c = (a & b) + '*'; char d = (a | b) - '-'; char e = (a ^ b) + '+'; printf("%c %c %c\n", c, d, e); return 0; }
(A) z K S
(B) 122 75 83
(C )∗ – +
(D) P x +
Ans: (A) z K S
Solution: char a = ‘P’
char b = ‘x’
As per precedence of operators, () evaluated prior to +/-
Note that &,^ and | are bit wise operators and Addition/Subtraction of characters applied on their ASCII values.
a = ‘P’ means a = ASCII value of (‘P’) = 65+15 = 80 = (0101 0000)2
b = ‘x’ means b = ASCII value of (‘x’) = 97+23 = 120 = (0111 1000)2
a & b = (0101 0000)2 [ apply & logic on corresponding bits ] = (80)10
‘*’ = 42 = (0010 1010)2
a&b + ‘*’ = 80 + 42 = 122
print character ( 122 ) = z
a | b = (0111 1000)2 [ apply | logic on corresponding bits ] = (120)10
‘-’ = 45
a|b – ‘-’ = 120 – 45 = 75
print character ( 75 ) = K
a ^ b = (0010 1000)2 [ apply ^ logic on corresponding bits ] = (40)10
‘+’ = 43
a^b + ‘+’ = 40 + 43 = 83
print character ( 83 ) = S