Error/Bug: Error is a mistake/bug in the program which makes the behavior of the program abnormal. It prevents a program from compiling and running correctly. So, the errors must be removed from the program for the successful execution of the program. Programming errors are also known as the bugs. These errors are detected either during the time of compilation or execution.
Debugging: The process of removing these bugs/errors is known as debugging. It refers to the process of locating the place of error, cause of error and correcting the code to produce the desired output.
Code Tracing: It refers to executing code one line at a time and watching its impact on the variables, to find the source of error.
Types of Errors: There are mainly two types of errors in Python.
- Compile Time Errors
a) Syntax error
b) Semantic error - Run Time errors:
a) Logical error
b) Exceptions
Compile Time Errors: These errors occur during the compilation time of the program.
Syntax Error: Syntax errors occur when a programmer makes mistakes in typing the code’s syntax correctly. In other words, syntax errors occur when a programmer does not follow the set of rules defined for the syntax of Python language. Syntax errors are sometimes also called Compile Time errors because they are always detected by the compiler/Interpreter. Generally, these errors can be easily identified and rectified by programmers. The most commonly occurring syntax errors in Python language are:
1. Uneven pair or Missing paranthesis ()
2. Uneven pair or Missing braces {}
3. Uneven pair or missing square bracket []
4. Wrong Indentation
5. Missing Colon : for a block
Let’s take an example:
a=5 print(a
Output:
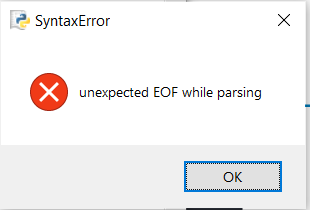
If we forget to write : at the end of a block, then also python gives syntax error as
>>> if 4>3 SyntaxError: invalid syntax
If we write the code without proper indentation, then also python gives syntax error as:
>>> print("hello") SyntaxError: unexpected indent
Semantic Error: Semantics refers to the set of rules which gives the meaning of a statement. These errors occur when the statements are not meaningful.
a=5 a + 2 = b
Output:
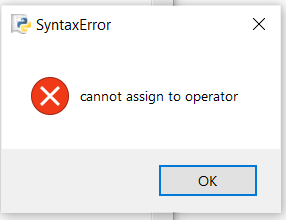
Run Time Errors: These errors occur during the execution or running of the program. These are harder to find. Example infinite loop, wrong input etc. They may stop the execution of the program.
Logical Error: When our program is error free, runs successfully, gives output, but the output is different from our expectations, then these types of errors are called Logical Errors. These errors are hardest to locate/find and remove because we have to check the whole program code very carefully and find the source of error, and then correct it.
a = 5 b = 7 sum= a * b print("sum of 5 and 7 is " , sum)
Output:
sum of 5 and 7 is 35
Exceptions: An exception is an event, which occurs during the execution of a program that disrupts the normal flow of the program’s instructions. It is an irregular situation that may occur during run time. We can check the built in Exceptions supported by Python as:
>>>#to count the no of built ins supported by python >>> len(dir(__builtins__)) 154 >>>#to display the built ins supported by python >>> dir(__builtins__) ['ArithmeticError', 'AssertionError', 'AttributeError', 'BaseException', 'BlockingIOError', 'BrokenPipeError', 'BufferError', 'BytesWarning', 'ChildProcessError', 'ConnectionAbortedError', 'ConnectionError', 'ConnectionRefusedError', 'ConnectionResetError', 'DeprecationWarning', 'EOFError', 'Ellipsis', 'EnvironmentError', 'Exception', 'False', 'FileExistsError', 'FileNotFoundError', 'FloatingPointError', 'FutureWarning', 'GeneratorExit', 'IOError', 'ImportError', 'ImportWarning', 'IndentationError', 'IndexError', 'InterruptedError', 'IsADirectoryError', 'KeyError', 'KeyboardInterrupt', 'LookupError', 'MemoryError', 'ModuleNotFoundError', 'NameError', 'None', 'NotADirectoryError', 'NotImplemented', 'NotImplementedError', 'OSError', 'OverflowError', 'PendingDeprecationWarning', 'PermissionError', 'ProcessLookupError', 'RecursionError', 'ReferenceError', 'ResourceWarning', 'RuntimeError', 'RuntimeWarning', 'StopAsyncIteration', 'StopIteration', 'SyntaxError', 'SyntaxWarning', 'SystemError', 'SystemExit', 'TabError', 'TimeoutError', 'True', 'TypeError', 'UnboundLocalError', 'UnicodeDecodeError', 'UnicodeEncodeError', 'UnicodeError', 'UnicodeTranslateError', 'UnicodeWarning', 'UserWarning', 'ValueError', 'Warning', 'WindowsError', 'ZeroDivisionError', '__build_class__', '__debug__', '__doc__', '__import__', '__loader__', '__name__', '__package__', '__spec__', 'abs', 'all', 'any', 'ascii', 'bin', 'bool', 'breakpoint', 'bytearray', 'bytes', 'callable', 'chr', 'classmethod', 'compile', 'complex', 'copyright', 'credits', 'delattr', 'dict', 'dir', 'divmod', 'enumerate', 'eval', 'exec', 'exit', 'filter', 'float', 'format', 'frozenset', 'getattr', 'globals', 'hasattr', 'hash', 'help', 'hex', 'id', 'input', 'int', 'isinstance', 'issubclass', 'iter', 'len', 'license', 'list', 'locals', 'map', 'max', 'memoryview', 'min', 'next', 'object', 'oct', 'open', 'ord', 'pow', 'print', 'property', 'quit', 'range', 'repr', 'reversed', 'round', 'set', 'setattr', 'slice', 'sorted', 'staticmethod', 'str', 'sum', 'super', 'tuple', 'type', 'vars', 'zip']
Description of some standard Exceptions:
Sr.No. | Exception | Description |
---|---|---|
1 | ArithmeticError | Raised when an error occurs in numeric calculations |
2 | EOFError | Raised when the input() method hits an “end of file” condition (EOF) |
3 | FloatingPointError | Raised when a floating point calculation fails |
4 | ImportError | Raised when an imported module does not exist |
5 | IndentationError | Raised when indentation is not correct |
6 | IndexError | Raised when an index of a sequence does not exist |
7 | KeyError | Raised when a key does not exist in a dictionary |
8 | MemoryError | Raised when a program runs out of memory |
9 | NameError | Raised when a variable does not exist |
10 | OSError | Raised when an operating system related operation causes an error |
11 | OverflowError | Raised when the result of a numeric calculation is too large |
12 | RecursionError | Raised when maximum recursion depth exceeded |
13 | SyntaxError | Raised when a syntax error occurs |
14 | TabError | Raised when indentation consists of tabs or spaces |
15 | TypeError | Raised when two different types are combined |
16 | ValueError | Raised when there is a wrong value in a specified data type |
17 | ZeroDivisionError | Raised when the second operator in a division is zero |
Examples of some standard Exceptions:
ArithmeticError: Raised when an error occurs in numeric calculations. Example:
>>> 50 / 0 Traceback (most recent call last): File "<pyshell#39>", line 1, in <module> 50 / 0 ZeroDivisionError: division by zero
ImportError: Raised when an imported module does not exist. Example:
>>> import academy Traceback (most recent call last): File "<pyshell#1>", line 1, in <module> import academy ModuleNotFoundError: No module named 'academy'
IndentationError: Raised when indentation is not correct. Example:
>>> a=5 >>> print(a) SyntaxError: unexpected indent
IndexError: Raised when an index of a sequence does not exist. Example:
>>> list1=[2,3,4,5] >>> list1[20] Traceback (most recent call last): File "<pyshell#1>", line 1, in <module> list1[20] IndexError: list index out of range
KeyError: Raised when a key does not exist in a dictionary. Example:
>>> dict1={1:"gargs", 2:"academy"} >>> dict1[5] Traceback (most recent call last): File "<pyshell#4>", line 1, in <module> dict1[5] KeyError: 5
NameError: Raised when a variable does not exist. Example
>>> print(message) Traceback (most recent call last): File "<pyshell#10>", line 1, in <module> print(message) NameError: name 'message' is not defined
OverflowError: Raised when the result of a numeric calculation is too large. Example
>>> import math >>> math.factorial(764537465743567) Traceback (most recent call last): File "<pyshell#9>", line 1, in <module> math.factorial(764537465743567) OverflowError: factorial() argument should not exceed 2147483647
RecursionError: Raised when maximum recursion depth exceeded. Example:
def a(): b() def b(): a() a()
Output:
File "C:/Users/DELL/AppData/Local/Programs/Python/Python39/garg.py", line 4, in b a() File "C:/Users/DELL/AppData/Local/Programs/Python/Python39/garg.py", line 2, in a b() RecursionError: maximum recursion depth exceeded
TypeError: Raised when two different types are combined or an inappropriate type value is given in a function. Example:
>>> "gargs academy" + 9467863365 Traceback (most recent call last): File "<pyshell#11>", line 1, in <module> "gargs academy" + 9467863365 TypeError: can only concatenate str (not "int") to str >>> import math >>> math.sqrt("garg") Traceback (most recent call last): File "<pyshell#13>", line 1, in <module> math.sqrt("garg") TypeError: must be real number, not str
ValueError: Raised when there is a wrong value in a specified data type. Example:
>>> a=int("garg") Traceback (most recent call last): File "<pyshell#15>", line 1, in <module> a=int("garg") ValueError: invalid literal for int() with base 10: 'garg' >>> a=float("gargs") Traceback (most recent call last): File "<pyshell#18>", line 1, in <module> a=float("gargs") ValueError: could not convert string to float: 'gargs'
ZeroDivisionError: Raised when the second operator in a division is zero. Example:
>>> 50 / 0 Traceback (most recent call last): File "<pyshell#39>", line 1, in <module> 50 / 0 ZeroDivisionError: division by zero