Text Files: In Python, a text file is a file that contains human-readable text. Text files are usually created with a text editor, and they can be read and written in Python using the built-in file handling functions.
1) They store information in the form of ASCII/Unicode characters.
2) Each line of text is terminated or delimited with a special character known as EOL (End of Line).
3) Some internal translations take place when EOL character is read or written.
4) In Python, default EOL character is ‘\n’ or ‘\r\n’
5) They store the text in same form as typed, and have extension .txt
6) They are also known as regular text files.
RollNoNameMarks 1Sheetal500 2Amit400 3Nidhi300 4Rajesh450 5Ansh350 file1.txt Regular text file
File Operations : We can perform various operations on a file such as
1) Opening the already existing file
2) Creating the new file
2) Writing data into file
3) Reading data from the file
4) Appending data into the file
5) Searching data into the file
6) Updating data into the file
7) Closing the file
File Object: It is a built-in object or link used to interact with a file on disk or in memory. A file object is created using the open() function, and provides methods and attributes for reading and writing data to the file. All operations are performed on files through the file object or file handle. To create a file object in Python, you can use the open() function, which takes two arguments: the name of the file, and the mode in which the file should be opened.
Opening Files: We can open files using the built-in function open(). The open() function takes two arguments: the name of the file you want to open, and the mode in which you want to open the file. Syntax is
file_object_name = open(“file name with complete path” , “file mode”)
File Mode: The file mode determines the purpose of opening the file i.e. whether the file is opened for reading, writing, appending, or some combination of these operations. The file mode is specified as the second argument to the open() function, and it is a string that contains one or more characters.
The most common file modes are:
- ‘r’ open for reading (default)
- ‘w’ open for writing, truncating the file first
- ‘x’ create a new file and open it for writing
- ‘a’ open for writing, appending to the end of the file if it exists
- ‘b’ binary mode
- ‘t’ text mode (default)
- ‘+’ open a disk file for updating (reading and writing)
- ‘U’ universal newline mode (deprecated)
Note:
1) Default file opening mode is “rt” which opens a text file for reading if file exists and gives error if file doesn’t exist.
2) In write mode, file is created if it doesn’t exist and overwritten if file already exists while “X” mode (exclusive creation) is used to create a new file and open it for writing. If the file already exists, the operation will fail and raise a FileExistsError. This mode is useful when you want to create a new file and avoid accidentally overwriting an existing file.
3) In append mode, file is created if it doesn’t exist and opened (but not ovrewritten) if file already exists and new contents are appended at the end of the file.
4) While opening files in read and write file modes, file pointer is placed at the begining of the file but in append mode, file pointer is placed at the end of the file.
5) We can write exactly any one of create/read/write/append mode i.e r/w/a/x mode at a time
6) We can’t have text and binary mode at the same time
7) ‘U’ mode is deprecated and will raise an exception in future versions of Python. It has no effect in Python 3.
8) We can combine any two or more possible combinations of file modes in any possible way by writing as
a) ‘rb’ , ‘br’ , ‘rt’ , ‘tr’ , ‘r+b’ , ‘rb+’ , ‘+rb’ , ‘b+r’ , ‘br+’ , ‘+br’
b) ‘wb’ , ‘bw’ , ‘wt’ , ‘tw’ , ‘w+b’ , ‘wb+’ , ‘+wb’ , ‘b+w’ , ‘bw+’ , ‘+bw’
c) ‘ab’ , ‘ba’ , ‘at’ , ‘ta’ , ‘a+b’ , ‘ab+’ , ‘+ab’ , ‘b+a’ , ‘ba+’ , ‘+ba’
d) ‘xb’ , ‘bx’ , ‘xt’ , ‘tx’ , ‘x+b’ , ‘xb+’ , ‘+xb’ , ‘b+x’ , ‘bx+’ , ‘+bx’
>>># default mode is "rt" i.e. open a text file for reading >>> f1=open("garg.txt") >>>#opening a text file for reading >>> f2=open("garg.txt" ,"r") >>>#opening a text file for writing >>> f3=open("garg.txt" ,"w") >>>#opening a text file for appending >>> f4=open("garg.txt" ,"a") >>>#will give error because file already exists, and it is used to avoid accidentally overwriting it. >>> f5=open("garg.txt" ,"x") Traceback (most recent call last): File "<pyshell#11>", line 1, in <module> f5=open("garg.txt" ,"x") FileExistsError: [Errno 17] File exists: 'garg.txt' >>>#error because file is opened for reading (default mode) and file doesn't exist >>> f6=open("gupta.txt") Traceback (most recent call last): File "<pyshell#12>", line 1, in <module> f6=open("gupta.txt") FileNotFoundError: [Errno 2] No such file or directory: 'gupta.txt' >>># We must give double slashes as it is a escape sequence and special meaning attached to character >>> f7=open("f:\demo\file1.txt") >>># We can write r to specify raw string in which single slashes are used >>># which means no special meaning attached to any character >>> f8=open(r"f:demofile1.txt") >>># no space allowed between r and file name >>> f9=open(r "f:demofile1.txt") SyntaxError: invalid syntax >>># opens a binary file in write mode but both reading and writing operations can be performed >>> f10=open("f:\demo\file1.dat", "+wb")
To avoid/handle FileNotFoundError (as in above case of opening file gupta.txt and assigning the link to file pointer f6) Exceptions, we must always open a file in read mode in try and except blocks. In this case, our program will not interrupt, but gives proper error message like “File not Found” or any other message specified by the user.
>>> try: f6=open("gupta.txt") except: print("File not Fund") File not Fund
Reading Text Files: After opening a file in read mode, we can use the following functions to read the contents of the file.
1) read() : to read the contents of the entire file and returns a string.
2) read(n) : to read the n number of bytes from the file or until EOF (End of File) occurs and returns a string.
3) readline() : to read 1 line from the file and returns a string.
4) readline(n) : to read n bytes from the current line or until EOL (End of Line) occurs and returns a string.
5) readlines() : to read all lines from the file and returns a list of strings.
Welcome to File Handling in Python There are 3 types of files 1. Text Files 2. Binary Files 3. Delimited Files file1.txt
>>> f1=open("f:\demo\file1.txt") >>> f1.read() 'Welcome to File Handling in PythonnThere are 3 types of filesn1. Text Filesn2. Binary Filesn3. Delimited Files' >>>#reads first 50 bytes from file >>> f2=open("f:\demo\file1.txt") >>> f2.read(50) 'thonnThere are 3 types of filesn1. Text Filesn2. B' >>> f3=open("f:\demo\file1.txt") >>> f3.readline() 'Welcome to File Handling in Pythonn' >>>#reads first 50 bytes from line >>> f4=open("f:\demo\file1.txt") >>> f4.readline(50) 'Welcome to File Handling in Pythonn' >>> f5=open("f:\demo\file1.txt") >>> f5.readlines() ['Welcome to File Handling in Pythonn', 'There are 3 types of filesn', '1. Text Filesn', '2. Binary Filesn', '3. Delimited Files'] >>># We can combine open() and read() methods as >>> open("f:\demo\file1.txt").read(10) 'Welcome to'
>>> f1=open("f:\demo\file1.txt") >>> # Method 1 >>> for line in f1.readlines(): print(line) >>> # Method 2 >>> for line in f1: print(line) >>># Method 3 >>> with open("f:\demo\file1.txt") as f1: for line in f1: print(line)
Welcome to File Handling in Python There are 3 types of files 1. Text Files 2. Binary Files 3. Delimited Files
The advantage of using with keyword for file handling in python is that it automatically closes a file after the block of the code, even if an exception or run time error occurs, before the end of the block. We need not close the file explicitly.
Removing whitespaces after reading file: After reading a file, newline characters are to be removed from the lines. We can remove them
1) By using strip() method with no argument or n as argument : It removes given character or whitespace from both sides of a string.
2) By using rstrip() method with no argument or n as argument It removes given character or whitespace from right side of a string.
3) By using replace() method : It replaces a given old character in a string with another specified character.
>>> f1=open("f:\demo\file1.txt") >>># Method 1 >>> for line in f1: print(line.strip()) >>># Method 2 >>> for line in f1: print(line.strip('n')) >>># Method 3 >>> for line in f1: print(line.rstrip()) >>># Method 4 >>> for line in f1: print(line.rstrip('n')) >>># Method 5 >>> for line in f1: print(line.replace('n',''))
Welcome to File Handling in Python There are 3 types of files 1. Text Files 2. Binary Files 3. Delimited Files
Closing Files: Once you are done reading from the file or performing other operations on it, you can use the close() method to close the file. It’s generally a good practice to close files , as leaving files open can cause problems with file locking, resource usage, and potential data corruption. In Python, files are automatically closed when the program terminates, but it’s still a good idea to close them explicitly to avoid any issues. We can close a file using the close() method. Syntax is:
file_object_name.close()
Example: f1.close()
It breaks the link of the file object and the file on the disk/memory. After that no task can be performed on that file.
Note: open() is a built in function but close() is a function of file handle object.
Program to print contents of a file line by line: We can write the program in both ways as
f1=open("f:\demo\file1.txt") lines=f1.readlines() for line in lines: print(line,end="") # We can also write as # print(line.strip()) # to remove leading and trailing spaces # otherwise a blank line will be displayed # after each line f1.close()
f1=open("f:\demo\file1.txt") for line in f1: print(line,end="") # We can also write as # print(line.strip()) # to remove leading and trailing spaces # otherwise a blank line will be displayed # after each line f1.close()
Outputs of above codes:
Welcome to File Handling in Python There are 3 types of files 1. Text Files 2. Binary Files 3. Delimited Files
Welcome to File Handling in Python There are 3 types of files 1. Text Files 2. Binary Files 3. Delimited Files
Writing into Text Files: After opening a file in write/append mode, we can use the following functions to write the contents into the file.
1) write(string) : to write the given string into the file.
2) writelines(list_of_strings) : to write the list of strings into the file.
>>> f1=open("f:\demo\file2.txt","w") >>> f1.write("hello students") 14 >>> f1.write("welcome to") 10 >>> f1.write("file handling") 13 >>> f1.write("in Python") 9 >>> f1.close() >>>
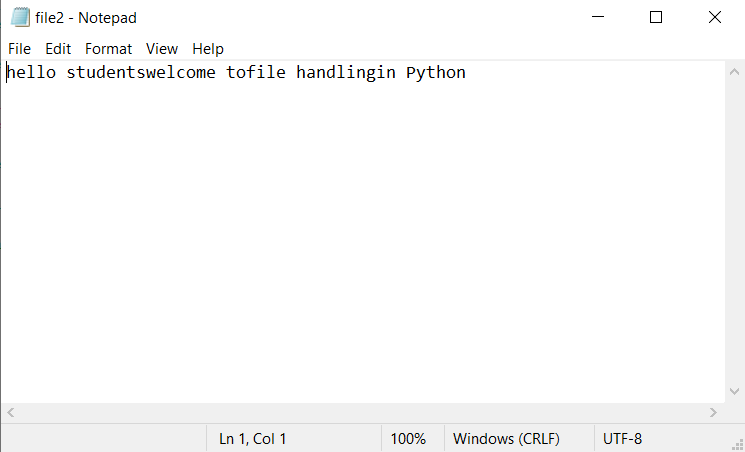
>>> f1=open("f:\demo\file2.txt","w") >>> l=[] >>> l.append("hello students") >>> l.append("welcome to") >>> l.append("file handling") >>> l.append("in python") >>> l ['hello students', 'welcome to', 'file handling', 'in python'] >>> f1.writelines(l) >>> f1.close() >>>
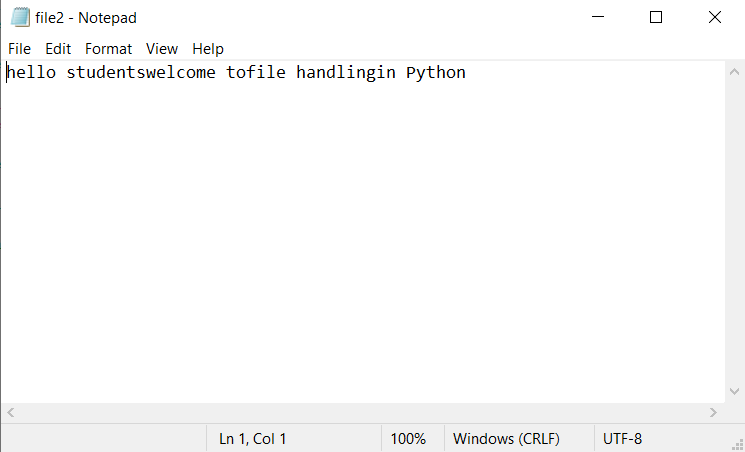
Both methods do not automatically add a EOL (End of Line) i.e. newline character (n) at the end of the string, So you need to add it manually if required. See the output of the following codes
>>> f1=open("f:\demo\file2.txt","w") >>> f1.write("hello students\n") 15 >>> f1.write("welcome to\n") 11 >>> f1.write("file handling\n") 14 >>> f1.write("in Python\n") 10 >>> f1.close()
>>> f1=open("f:\demo\file2.txt","w") >>> l=[] >>> l.append("hello students\n") >>> l.append("welcome to\n") >>> l.append("file handling\n") >>> l.append("in python\n") >>> f1.writelines(l) >>> f1.close()
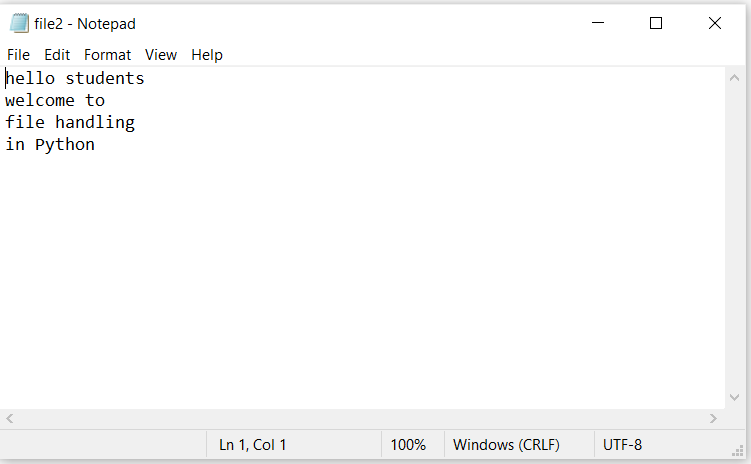
flush(): It forces the writing of data (still pending in output buffer) to be written into the file on disk/memory. Syntax is:
file_object.flush()
Example:
f1.write(“hello”)
f1.write(“students”)
f1.flush()
Note: These two lines i.e. “hello” and “students” are in output buffer and will be written to the file (pointed to by the file object f1) only after flush() or close() function. If we don’t want to close the file, but want to write these lines into the file, we use flush() function.
Standard Input / Output / Error Streams: Our standard devices
1) Standard input device (stdin) : keyboard
2) Standard output device (stdout) : monitor
3) Standard error device (stderr) : monitor
are implemented as files called standard streams.
These streams are defined in sys module and so we need to import sys module to use any of these file streams.
We can print the contents/string of a file on output stream or error stream.
>>> import sys >>> f1=open("f:\demo\file1.txt") >>> l1=f1.readline() >>> sys.stdout.write(l1) Welcome to File Handling in Python 35 >>> sys.stderr.write("Successful") Successful10