Error/Bug: Error is a mistake/bug in the program which makes the behavior of the program abnormal. So, the errors must be removed from the program for the successful execution of the program. Programming errors are also known as the bugs. These errors are detected either during the time of compilation or execution.
Debugging: The process of removing these bugs/errors is known as debugging.
Types of Errors: There are mainly four types of errors in C programming.
- Syntax error
- Semantic error
- Run-time error
- Logical error
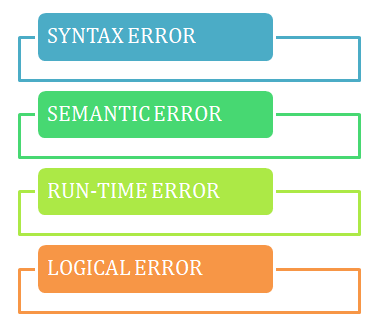
Syntax Error: Syntax errors occur when a programmer makes mistakes in typing the code’s syntax correctly. In other words, syntax errors occur when a programmer does not follow the set of rules defined for the syntax of C language.
Syntax errors are sometimes also called compilation errors because they are always detected by the compiler. Generally, these errors can be easily identified and rectified by programmers. The most commonly occurring syntax errors in C language are:
- Missing semi-colon (;)
- Missing paranthesis({})
- Assigning value to a variable without declaring it
Let’s take an example:
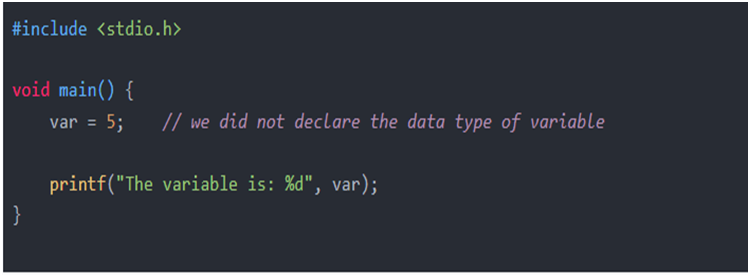
Output:

Semantic Error: Errors that occur because the compiler is unable to understand the written code are called Semantic Errors. A semantic error will be generated if the code makes no sense to the compiler, even though it is syntactically correct. It is like using the wrong word in the wrong place in the English language. For example, adding a string to an integer will generate a semantic error.
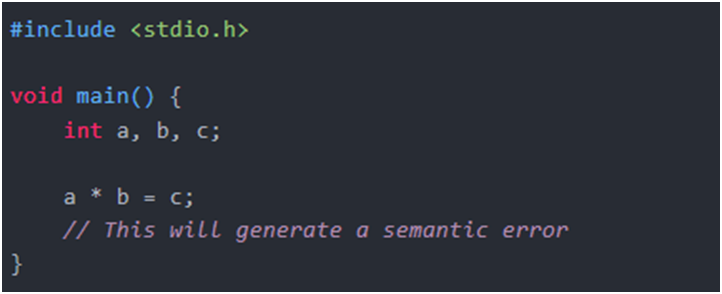

When we have an expression on the left hand side of an assignment operator (=), the program generates a semantic error. Even though the code is syntactically correct, the compiler does not understand the code.
Run Time Error: Errors that occur during the execution (or running) of a program are called Run Time Errors. These errors occur after the program has been compiled successfully. When a program is running, and it is not able to perform any particular operation, it means that we have encountered a run time error. Run time errors can be a little tricky to identify because the compiler cannot detect these errors. They can only be identified once the program is running. Some of the most common run time errors are: number not divisible by zero, array index out of bounds, string index out of bounds, etc. Run time errors can occur because of various reasons. Some of the reasons are:
- Mistakes in the Code: Let us say during the execution of a while loop, the programmer forgets to enter a break statement. This will lead the program to run infinite times, hence resulting in a run time error.
- Memory Leaks: If a programmer creates an array in the heap but forgets to delete the array’s data, the program might start leaking memory, resulting in a run time error.
- Mathematically Incorrect Operations: Dividing a number by zero, or calculating the square root of -1 will also result in a run time error.
- Undefined Variables: If a programmer forgets to define a variable in the code, the program will generate a run time error.
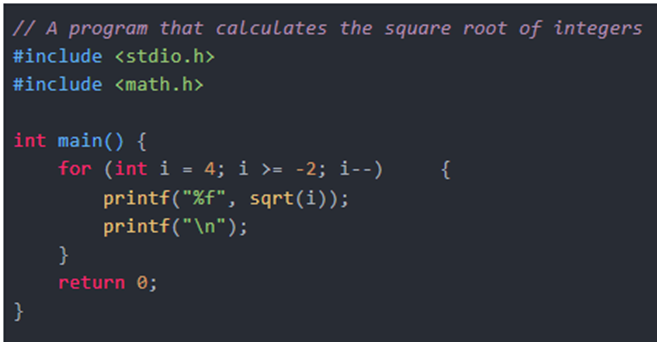
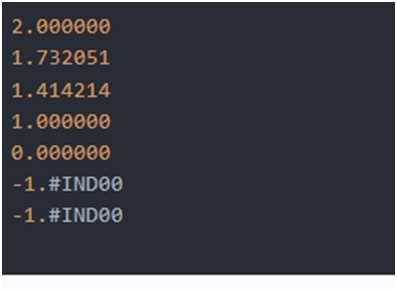
Logical Error: Sometimes, we do not get the output we expected after the compilation and execution of a program. Even though the code seems error free, the output generated is different from the expected one. These types of errors are called Logical Errors. Logical errors are those errors in which we think that our code is correct, the code compiles without any error and gives no error while it is running, but the output we get is different from the output we expected.
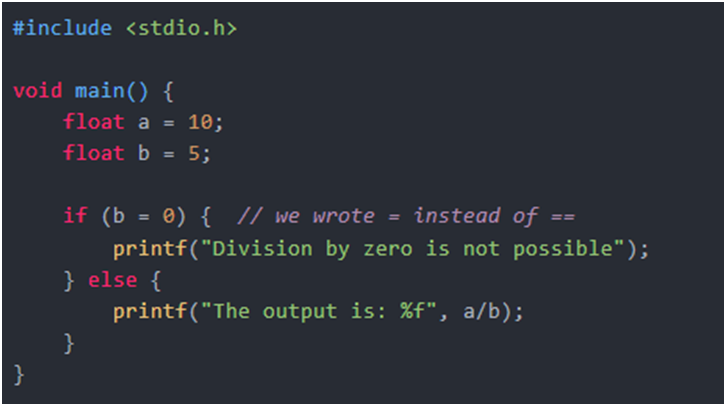
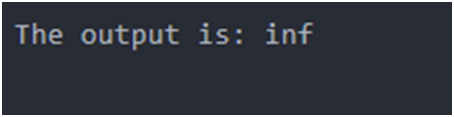
INF signifies a division by zero error. In the above example, at line 8, we wanted to check whether the variable b was equal to zero. But instead of using the equal to comparison operator (==), we use the assignment operator (=). Because of this, the if statement became false and the value of b became 0. Finally, the else clause got executed.